Overview
Learn how to retrieve and connect to the real-time matches data feed using the WebSockets API.
This guide will help you to retrieve matches that support real-time data and connect to the dedicated WebSockets.
Connecting to WebSockets
1. Retrieving matches that support real-time data
Support for real-time data is enabled at the tournament level. When available, the tournament live_supported
is true
.
For all children matches in the tournament, a WebSocket is opened 15 minutes prior to the scheduled playing time.
At any time, you can use the All Video Games > List live matches to get the list of currently opened WebSockets.
This endpoint returns a list of matches. Each match has a structure similar to the example below.
{
"endpoints": [
{
"begin_at": null,
"expected_begin_at": "2021-08-13T11:34:59Z",
"last_active": null,
"match_id": 595477,
"open": true,
"type": "frames",
"url": "wss://live.pandascore.co/matches/595477"
},
{
"begin_at": null,
"expected_begin_at": "2021-08-13T11:34:59Z",
"last_active": null,
"match_id": 595477,
"open": true,
"type": "events",
"url": "wss://live.pandascore.co/matches/595477/events"
}
],
"match": {
// Omitted for clarity
}
}
2. Connecting to the real-time feeds
The previous JSON contains two endpoints: a Frames endpoint and an Events endpoint.
Using your preferred application or language, you can perform a secure connection to the WebSockets. Don't forget to include your token as a token
URL parameter.
wscat -c 'wss://live.pandascore.co/matches/595477?token=YOUR_TOKEN'
const socket = new WebSocket('wss://live.pandascore.co/matches/8191?token=YOUR_TOKEN')
socket.onmessage = function (event) {
console.log(JSON.parse(event.data))
}
wscat is a command-line utility to connect to and display messages from WebSockets.
After a successful connection, the server sends a hello event as below.
{"type":"hello","payload":{}}
Users are allowed a maximum of 3 simultaneous connections per match per endpoint.
In case of disconnects, see Disconnections.
Frames & Events
PandaScore WebSockets API offers two real-time data feeds:
- Frames — snapshot of all the game data points, taken every 2 seconds
- Events — timeline of events happening in game, sent as they occur.
Frames feed
All real-time integrations start with the frames feed. As the frames data contains all team statistics, it is often crucial to have it. In fact, it makes handling the events easier.
Using the frames feed, you can create real-time scoreboard like below.
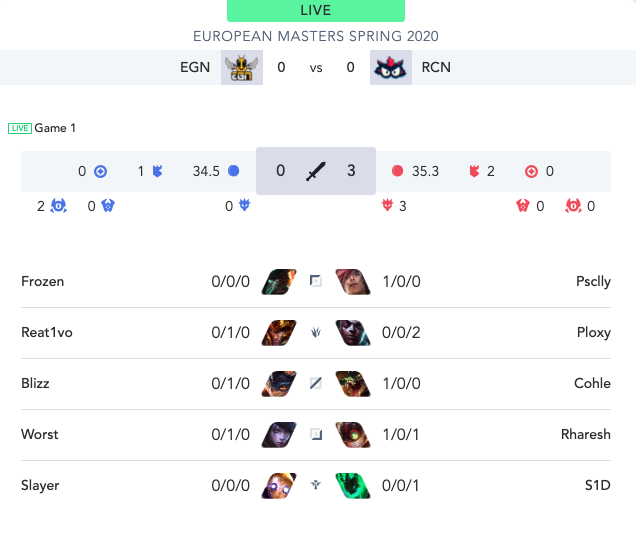
Example League of Legends scoreboard
For League of Legends, frames can also be retrieved after the game with the LoL > List game play-by-play frames endpoint. For Counter-Strike, rounds can be retrieved after the game with the CS > List game play-by-play rounds endpoint.
Events feed
Notice
The events feed is available in the Live Pro Plan. For more information, see Pricing
On the other side, events represent a timeline of the game. Events are sent for major actions happening in game such as kills, Baron kills (in League), or the bomb exploding (in CS:GO).
Using the events feed, you can create real-time timelines like below.

Example Counter-Strike timeline
It is also possible to request a recap of all past events using Events recovery.
For League of Legends and Counter-Strike, Events can also be retrieved after the game with the LoL > List game play-by-play events and CS > List game play-by-play events endpoints.
Updated over 1 year ago